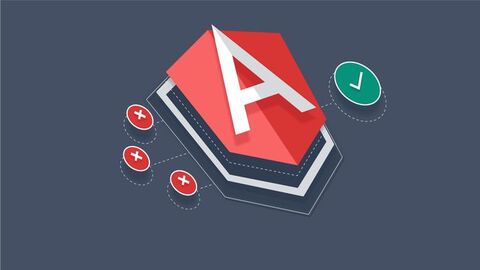
Securing Your Angular App: The Crucial Role of Authentication Guards
May 1, 2024
Introduction to Angular Authentication Guards:
Angular Authentication Guards serve as gatekeepers for your application, regulating access based on user authentication status. They are essential components of Angular security, ensuring that only authorized users can access protected routes and resources. By understanding and implementing authentication guards effectively, you can fortify your application against unauthorized access and potential security threats.
Understanding the Importance of Authentication in Web Applications:
Authentication forms the bedrock of web application security, verifying the identity of users and controlling access to sensitive resources. Inadequate authentication mechanisms can lead to data breaches and unauthorized access, compromising the integrity of the application and user privacy. Understanding the critical role of authentication underscores the importance of implementing robust authentication measures, including authentication guards, to safeguard your Angular application.
Exploring Angular’s Built-in Authentication Mechanisms:
Angular provides a comprehensive suite of built-in authentication mechanisms, empowering developers to implement authentication seamlessly. These mechanisms include route guards, interceptors, and authentication services, offering a versatile toolkit for securing Angular applications. By exploring and leveraging Angular’s native authentication features, developers can streamline the implementation process and enhance the overall security posture of their applications.
The Fundamentals: Setting Up Authentication Guards in Angular:
Setting up authentication guards in Angular involves defining guard services that implement Angular’s `CanActivate`, `CanActivateChild`, `CanLoad`, or `CanDeactivate` interfaces. These guard services contain logic to determine whether a user is authorized to access specific routes or perform certain actions within the application. By mastering the fundamentals of setting up authentication guards, developers can establish a solid foundation for implementing robust security measures in their Angular applications.
Leveraging Route Guards for Secure Navigation:
Route guards play a pivotal role in controlling navigation within Angular applications based on the authentication state of the user. By leveraging route guards effectively, developers can enforce access restrictions to certain routes, redirect users to login pages when necessary, or prevent unauthorized navigation altogether. Implementing secure navigation with route guards enhances the overall security of Angular applications by ensuring that sensitive areas are accessible only to authenticated users.
Implementing Role-Based Access Control with Authentication Guards:
Role-based access control (RBAC) is a fundamental security concept that involves assigning permissions to users based on their roles within an organization. Authentication guards in Angular can be customized to enforce RBAC policies, allowing developers to restrict access to certain functionalities or resources based on the user’s role. By implementing RBAC with authentication guards, developers can ensure that users only have access to the features and data that are relevant to their roles, enhancing security and maintaining data integrity.
Handling Authentication Errors and Redirects Effectively:
Effective error handling and redirection are essential aspects of user experience and security in Angular applications. Authentication guards can be used to detect authentication errors, such as expired or invalid tokens, and redirect users to appropriate error pages or login screens. By handling authentication errors gracefully, developers can provide users with clear feedback and guidance, improving overall usability and security.
Advanced Techniques: Customizing Authentication Guards for Complex Scenarios:
In complex application scenarios, standard authentication guard implementations may not suffice. Advanced customization techniques, such as combining multiple guards, asynchronous validation, or integrating with external authentication providers, may be required. By mastering advanced customization techniques, developers can address the unique security requirements of their applications and ensure robust protection against various security threats.
Protecting Lazy Loaded Modules with Authentication Guards:
Lazy loading is a technique used to improve the performance of Angular applications by loading modules asynchronously. However, it’s essential to ensure that lazy-loaded modules are protected from unauthorized access. Authentication guards can be applied to lazy-loaded routes to enforce authentication and authorization requirements, ensuring that only authenticated users can access these modules.
Securing HTTP Requests with Interceptors and Guards:
Secure communication between Angular applications and backend servers is critical for protecting sensitive data. Interceptors and guards can be used together to intercept HTTP requests, attach authentication tokens, and enforce security policies before sending requests to the server. By securing HTTP requests, developers can prevent unauthorized access to backend resources and mitigate various security risks, such as data tampering or injection attacks.
Implementing Token-based Authentication in Angular:
Token-based authentication is a widely used approach for securing web applications, including those built with Angular. This section will explore the implementation of token-based authentication in Angular applications, covering concepts such as token generation, storage, and validation. By implementing token-based authentication, developers can enhance the security of their Angular applications by securely managing user sessions and verifying user identities.
Integrating Third-Party Authentication Providers with Angular:
Integrating third-party authentication providers, such as OAuth or social login services, with Angular applications can streamline the user authentication process and provide a seamless user experience. This section will delve into the process of integrating third-party authentication providers with Angular, covering authentication flows, configuration, and best practices. By leveraging third-party authentication providers, developers can offer users convenient authentication options while maintaining the security of their applications.
Enhancing Security with Multi-factor Authentication (MFA):
Multi-factor authentication (MFA) adds an extra layer of security to Angular applications by requiring users to provide multiple forms of verification before gaining access. This section will explore the implementation of MFA in Angular applications, covering methods such as SMS verification, email verification, and authenticator apps. By implementing MFA, developers can significantly reduce the risk of unauthorized access and strengthen the overall security posture of their applications.
Scaling Authentication: Strategies for Large Angular Applications:
As Angular applications grow in complexity and user base, scaling authentication becomes increasingly challenging. This section will discuss strategies for scaling authentication in large Angular applications, covering topics such as distributed authentication, session management, and performance optimization. By implementing scalable authentication strategies, developers can ensure that their Angular applications can handle large volumes of users while maintaining security and performance.
Caching Authentication State for Improved Performance:
Caching authentication state can significantly improve the performance of Angular applications by reducing the need to repeatedly authenticate users on every request. This section will explore techniques for caching authentication state in Angular applications, covering concepts such as session caching, token caching, and cache invalidation strategies. By implementing efficient authentication state caching mechanisms, developers can enhance the responsiveness and scalability of their Angular applications.
Extending Angular’s Security: Cross-Site Request Forgery (CSRF) Protection:
Cross-Site Request Forgery (CSRF) attacks pose a significant threat to web applications, including those built with Angular. This section will discuss techniques for protecting Angular applications against CSRF attacks, covering concepts such as CSRF tokens, SameSite cookies, and CSRF protection headers. By implementing robust CSRF protection measures, developers can prevent malicious actors from exploiting vulnerabilities in their Angular applications and safeguard user data.
Auditing and Monitoring Authentication Events in Angular Apps:
Auditing and monitoring authentication events are essential for detecting and responding to security incidents in Angular applications. This section will explore techniques for auditing and monitoring authentication events, covering topics such as logging authentication events, analyzing authentication logs, and implementing real-time monitoring solutions. By establishing comprehensive auditing and monitoring mechanisms, developers can identify and mitigate security threats in their Angular applications proactively.
Best Practices for Maintaining Security with Angular Authentication Guards:
This section will summarize best practices for maintaining security with Angular authentication guards, covering topics such as secure authentication workflows, user session management, and secure storage of authentication tokens. By following best practices, developers can ensure that their Angular applications are resilient to security threats and provide users with a secure and seamless authentication experience.