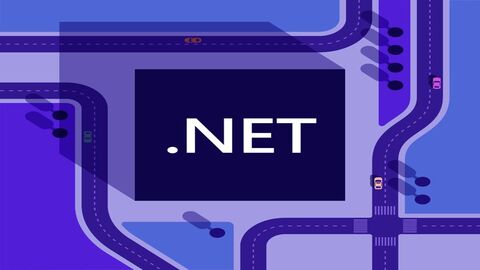
Authentication and Authorization in .NET Core
April 24, 2024
Introduction to Authentication and Authorization:
Authentication and authorization are fundamental aspects of modern application security. Authentication is the process of verifying the identity of a user, ensuring they are who they claim to be. This typically involves presenting credentials such as a username and password, tokens, or biometric data. Once authenticated, authorization determines what actions a user is allowed to perform within the application based on their identity and associated permissions or roles. Together, authentication and authorization form the basis for controlling access to resources and ensuring data security.
Understanding .NET Core Security Fundamentals:
.NET Core provides a comprehensive set of security features to help developers build secure applications. These include built-in mechanisms for authentication, authorization, data protection, and secure communication. Understanding these fundamentals is crucial for implementing effective security measures in .NET Core applications. Developers need to be familiar with concepts such as identity management, cryptographic algorithms, secure communication protocols, and best practices for protecting sensitive data.
Configuring Authentication in .NET Core:
Configuring authentication in .NET Core involves setting up the mechanisms through which users can prove their identity to the application. This includes choosing authentication schemes such as cookies, tokens, or external providers like OAuth and OpenID Connect. Developers need to understand the available options and choose the appropriate authentication method based on their application’s requirements and security considerations. Configuration involves specifying authentication middleware, defining authentication options, and integrating with external identity providers if necessary.
Implementing User Authentication with ASP.NET Core Identity:
ASP.NET Core Identity is a membership system that adds login functionality to ASP.NET Core applications. It provides features for managing user accounts, including registration, login, password recovery, and role-based authorization. Implementing user authentication with ASP.NET Core Identity involves configuring the Identity framework, creating user interfaces for registration and login, and integrating it with the application’s authentication pipeline. Developers can customize the user model, implement custom password policies, and extend identity management functionality as needed.
Customizing Identity Management in ASP.NET Core:
While ASP.NET Core Identity offers a robust set of features out of the box, developers often need to customize it to fit their specific requirements. This may involve extending the user model to include additional fields or properties, implementing custom password policies or validation rules, integrating with external identity providers, or changing the default UI elements to match the application’s branding. Customizing identity management allows developers to tailor the authentication experience to their application’s needs while maintaining security and compliance requirements.
Working with External Authentication Providers (OAuth, OpenID Connect):
External authentication providers such as OAuth and OpenID Connect enable users to authenticate using their existing accounts from third-party services like Google, Facebook, or Microsoft. Integrating external authentication providers in a .NET Core application involves configuring the authentication middleware, handling callback requests, and mapping external user identities to local user accounts. This approach simplifies the authentication process for users and allows developers to leverage the security features provided by established identity providers.
Securing APIs with JWT (JSON Web Tokens) Authentication:
JSON Web Tokens (JWT) are a popular choice for securing APIs due to their compact format, self-contained nature, and support for claims-based authentication. In .NET Core, JWT authentication involves issuing tokens to authenticated users, validating incoming tokens, and extracting claims to authorize access to API endpoints. Implementing JWT authentication requires careful consideration of token generation, validation, and revocation mechanisms to ensure the security and integrity of the authentication process.
Role-Based Access Control (RBAC) in .NET Core:
Role-Based Access Control (RBAC) is a widely used authorization model that assigns permissions to users based on their roles within an organization. In .NET Core, RBAC can be implemented using built-in features such as ASP.NET Core Identity roles or custom role management solutions. Managing roles, assigning permissions, and enforcing access control based on user roles are essential aspects of implementing RBAC in .NET Core applications. RBAC provides a flexible and scalable approach to access control, allowing administrators to define granular permissions based on user roles and responsibilities.
Policy-Based Authorization in ASP.NET Core:
Policy-Based Authorization in ASP.NET Core provides a flexible and declarative way to define access control rules based on various criteria such as user roles, claims, or custom conditions. Policies are defined centrally and can be applied to controllers, actions, or Razor Pages using authorization attributes. Implementing policy-based authorization involves defining authorization policies, applying them to endpoints, and handling access denied scenarios. This approach allows developers to implement complex authorization requirements using a rule-based approach, making it easier to manage and maintain authorization logic across the application.
Implementing Custom Authorization Handlers:
Custom authorization handlers in .NET Core allow developers to implement fine-grained access control logic beyond what is possible with built-in authorization features. Custom handlers can inspect incoming requests, evaluate complex authorization requirements, and make authorization decisions based on custom criteria. Implementing custom authorization handlers involves creating classes that implement the `IAuthorizationHandler` interface and registering them in the application’s authorization pipeline. This approach enables developers to implement specialized authorization logic tailored to the specific requirements of their application, enhancing security and flexibility.
IdentityServer4 Integration for Centralized Authentication and Authorization:
IdentityServer4 is an open-source framework that provides authentication and authorization services for .NET Core and ASP.NET Core applications. It allows developers to centralize authentication and authorization logic, support single sign-on (SSO) across multiple applications, and implement standards-based protocols such as OAuth 2.0 and OpenID Connect. Integrating IdentityServer4 involves configuring clients, defining scopes, and protecting APIs and resources using access tokens. This approach provides a scalable and extensible solution for managing authentication and authorization in distributed systems, improving security and user experience.
Multi-Factor Authentication (MFA) in .NET Core Applications:
Multi-Factor Authentication (MFA) adds an extra layer of security to .NET Core applications by requiring users to verify their identity using multiple factors such as passwords, biometrics, or one-time codes. Implementing MFA involves integrating with authentication providers that support MFA, implementing verification methods, and enforcing MFA policies based on user preferences or security requirements. MFA helps mitigate the risk of unauthorized access by requiring additional verification steps beyond traditional password-based authentication, enhancing security and protecting sensitive data.
Token-Based Authentication for SPA (Single Page Application) with .NET Core:
Single Page Applications (SPAs) often use token-based authentication to secure access to APIs and resources. In .NET Core, token-based authentication typically involves issuing JWT tokens to authenticated users and validating them on the server side. Implementing token-based authentication for SPAs requires setting up authentication endpoints, implementing token generation and validation logic, and handling token expiration and renewal. This approach provides a stateless authentication mechanism that is well-suited for distributed and scalable web applications, improving security and performance.
Securing SignalR Applications in .NET Core:
SignalR is a real-time communication library for .NET Core that enables bi-directional communication between clients and servers over WebSockets or other transport mechanisms. Securing SignalR applications involves implementing authentication and authorization mechanisms to control access to hubs and hub methods. This may include integrating with ASP.NET Core Identity, using JWT tokens for authentication, or implementing custom authentication handlers. Securing SignalR applications helps prevent unauthorized access and ensures the integrity and confidentiality of real-time data exchanged between clients and servers.
Securing Microservices with .NET Core:
Microservices architecture involves breaking down large monolithic applications into smaller, independently deployable services. Securing microservices in .NET Core requires implementing authentication and authorization mechanisms that work across service boundaries. This may involve using OAuth 2.0 for authentication, JWT tokens for authorization, and service-to-service authentication using client credentials or service principals. Securing microservices helps protect sensitive data and prevent unauthorized access to critical resources, ensuring the overall security and integrity of the distributed system.
Best Practices for Secure Authentication and Authorization:
Implementing secure authentication and authorization requires following best practices to mitigate common security risks such as authentication bypass, session hijacking, and privilege escalation. Best practices include using strong cryptographic algorithms, protecting sensitive data, enforcing least privilege access, and regularly auditing and monitoring authentication and authorization events. By following best practices, developers can build resilient and secure applications that protect against a wide range of security threats and vulnerabilities, ensuring the confidentiality, integrity, and availability of sensitive information.
Testing Authentication and Authorization in .NET Core Applications:
Testing authentication and authorization logic is essential to ensure that security measures are correctly implemented and effective against potential threats. In .NET Core, testing authentication and authorization involves writing unit tests to verify behavior under different authentication and authorization scenarios, including authenticated and unauthenticated users, users with different roles and permissions, and invalid authentication attempts. Automated testing helps identify security vulnerabilities and ensures that authentication and authorization mechanisms function as intended, enhancing the overall security posture of the application.
Advanced Security Considerations and Threat Mitigation in .NET Core Applications:
Advanced security considerations and threat mitigation strategies are necessary to address evolving security threats and vulnerabilities in .NET Core applications. This includes techniques such as input validation, output encoding, secure communication, secure configuration management, and mitigations against common attacks such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Implementing these measures helps developers build resilient and secure applications that protect against a wide range of security risks, ensuring the confidentiality, integrity, and availability of sensitive information. By adopting advanced security practices, developers can proactively identify and mitigate security threats, reducing the risk of security breaches and data leaks.