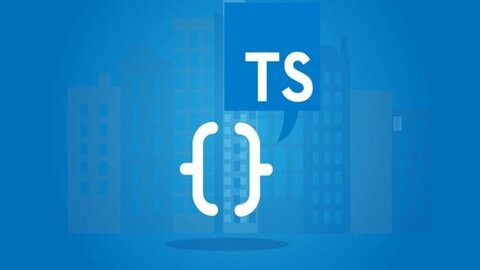
Advanced TypeScript Features: Generics, Decorators, and Modules
April 4, 2024
Introduction to Advanced TypeScript Concepts
In this chapter, we provide a comprehensive overview of the advanced TypeScript features covered in this book: Generics, Decorators, and Modules. We discuss their significance in modern TypeScript development and how they contribute to writing cleaner, more maintainable, and scalable codebases. By understanding these concepts, developers gain the ability to tackle complex challenges and architect robust solutions effectively. Through real-world examples and use cases, we illustrate how mastering Generics, Decorators, and Modules empowers developers to build sophisticated applications with confidence. This introductory chapter sets the stage for an in-depth exploration of these essential topics, laying the foundation for readers to grasp the advanced TypeScript concepts discussed throughout the book.
Understanding TypeScript Generics
Generics are a powerful feature in TypeScript that enables writing reusable and flexible code by parameterizing types. In this chapter, we delve into the fundamentals of generics, exploring how they facilitate the creation of functions, classes, and interfaces that work with a variety of data types. We discuss common patterns and best practices for using generics effectively, along with practical examples to demonstrate their utility in different scenarios. By mastering generics, developers gain the ability to write more generic and adaptable code that can handle a wide range of input types without sacrificing type safety or code clarity.
Advanced Generics: Constraints and Conditional Types
Building upon the basics, we explore advanced techniques for working with generics in TypeScript. This includes understanding type constraints, which allow developers to impose specific requirements on the types used with generics, enhancing type safety and expressiveness. Additionally, we delve into conditional types, a powerful feature that enables creating complex type transformations based on conditional logic. Through in-depth explanations and hands-on examples, developers will learn how to leverage these advanced generics features to solve sophisticated problems and write more expressive and type-safe code.
Using Generics in Function Overloads
Function overloading is a technique used to define multiple function signatures for a single function, allowing it to accept different combinations of argument types. In this chapter, we explore how generics can be seamlessly integrated with function overloads to create highly flexible and polymorphic functions. By understanding the interplay between generics and function overloads, developers can design APIs that cater to a diverse set of use cases while maintaining clarity and type safety. Through practical examples and best practices, readers will learn how to harness the power of generics to write versatile and reusable functions that adapt to various input scenarios.
Mastering Type Inference with Generics
TypeScript’s powerful type inference capabilities enable the compiler to deduce types based on usage context, reducing the need for explicit type annotations and improving developer productivity. In this chapter, we explore how generics can enhance type inference, allowing TypeScript to automatically infer complex types based on usage patterns. By leveraging generics to guide type inference, developers can write concise and expressive code without sacrificing type safety or readability. Through real-world examples and best practices, readers will learn how to harness the full potential of TypeScript’s type inference engine to write more maintainable and expressive codebases.
Exploring Decorators: Basics and Syntax
Decorators are a feature in TypeScript that allows developers to add metadata and behavior to class declarations and members. In this chapter, we provide a comprehensive overview of decorators, covering their syntax, usage, and common use cases. We explore how decorators can be applied to classes, methods, properties, and parameters, enabling developers to implement cross-cutting concerns such as logging, validation, and dependency injection. By understanding the basics of decorators, developers gain a powerful tool for enhancing code readability, maintainability, and extensibility. Through practical examples and best practices, readers will learn how to leverage decorators effectively to streamline development workflows and build robust and modular applications.
Working with Class Decorators
Class decorators are a fundamental building block of TypeScript’s decorator system, allowing developers to apply behavior to classes at design time. In this chapter, we delve deeper into class decorators, exploring their usage patterns, lifecycle hooks, and common design patterns. We discuss how class decorators can be used to implement advanced features such as dependency injection, aspect-oriented programming, and domain-specific languages. By mastering class decorators, developers gain a powerful tool for extending and customizing the behavior of classes in a flexible and composable manner. Through hands-on examples and best practices, readers will learn how to harness the full potential of class decorators to build modular and extensible software architectures.
Understanding Property Decorators
Property decorators allow developers to add metadata and behavior to class properties in TypeScript, enhancing their expressiveness and flexibility. In this chapter, we explore the different types of property decorators, including accessor, method, and parameter decorators, along with their common use cases. We discuss how property decorators can be used to implement features such as validation, memoization, lazy initialization, and access control. By understanding the nuances of property decorators, developers gain a powerful tool for enhancing the behavior and semantics of class properties. Through practical examples and best practices, readers will learn how to leverage property decorators effectively to build more expressive and maintainable codebases.
Method Decorators: Extending Functionality
Method decorators provide a mechanism for adding behavior to class methods in TypeScript, enabling developers to implement cross-cutting concerns such as logging, caching, and access control. In this chapter, we explore how method decorators can be used to enhance the behavior of class methods in a flexible and composable manner. We discuss common use cases and design patterns for method decorators, including how to handle method parameters and return values. By mastering method decorators, developers gain a powerful tool for extending the functionality of class methods without modifying their implementation. Through real-world examples and best practices, readers will learn how to leverage method decorators effectively to build modular and extensible software architectures.
Parameter Decorators: Advanced Use Cases
Parameter decorators allow developers to add behavior to function parameters in TypeScript, enabling features such as validation, dependency injection, and aspect-oriented programming. In this chapter, we explore advanced use cases for parameter decorators, including how to implement custom parameter transformations and how to handle variadic function parameters. We discuss common design patterns and best practices for parameter decorators, including how to handle optional and default parameter values. By mastering parameter decorators, developers gain a powerful tool for enhancing the behavior of functions in a flexible and composable manner. Through hands-on examples and best practices, readers will learn how to leverage parameter decorators effectively to build modular and extensible software architectures.
Decorator Factories: Dynamic Decorator Creation
Decorator factories allow developers to create dynamic decorators with configurable behavior in TypeScript, enabling features such as dependency injection, cross-cutting concerns, and aspect-oriented programming. In this chapter, we explore how decorator factories can be used to implement reusable and composable decorator patterns. We discuss common design patterns and best practices for decorator factories, including how to handle decorator parameters and how to compose multiple decorators. By mastering decorator factories, developers gain a powerful tool for building modular and extensible software architectures. Through real-world examples and best practices, readers will learn how to leverage decorator factories effectively to streamline development workflows and enhance code maintainability.
Advanced Techniques with Decorators
Building upon the previous chapters, we explore advanced techniques and patterns for working with decorators in TypeScript, including how to compose decorators, how to handle decorator execution order, and how to integrate decorators with other TypeScript features such as generics and conditional types. We discuss common
design patterns and best practices for building reusable and composable decorator patterns, including how to handle decorator parameters and how to create dynamic decorator factories. By mastering advanced techniques with decorators, developers gain a deeper understanding of how to leverage decorators effectively to build modular and extensible software architectures. Through hands-on examples and best practices, readers will learn how to harness the full potential of decorators to streamline development workflows and enhance code maintainability.
Understanding TypeScript Modules
Modules are a fundamental concept in modern JavaScript and TypeScript development, providing a way to organize code into reusable and maintainable units. In this chapter, we provide a comprehensive overview of TypeScript modules, covering their syntax, usage, and common use cases. We discuss the differences between ES6 modules and TypeScript modules, including how to export and import values, how to organize module files, and how to handle module dependencies. By understanding the basics of TypeScript modules, developers gain a powerful tool for building modular and maintainable codebases. Through practical examples and best practices, readers will learn how to leverage TypeScript modules effectively to streamline development workflows and enhance code maintainability.
Organizing Code with Namespaces and Modules
Namespaces and modules are two complementary mechanisms for organizing code in TypeScript, providing a way to encapsulate and isolate code into reusable and maintainable units. In this chapter, we explore the differences between namespaces and modules, covering their syntax, usage, and common use cases. We discuss best practices for organizing large-scale applications using namespaces and modules, including how to structure module files, how to manage module dependencies, and how to handle cross-module communication. By understanding the nuances of namespaces and modules, developers gain a powerful tool for building modular and maintainable codebases. Through real-world examples and best practices, readers will learn how to leverage namespaces and modules effectively to streamline development workflows and enhance code maintainability.
Deep Dive into ES6 Modules and TypeScript
ES6 modules are a standardized module system for JavaScript, widely supported by modern browsers and Node.js. In this chapter, we explore how TypeScript leverages ES6 modules, covering their syntax, usage, and common use cases. We discuss how to export and import values using ES6 modules, how to organize module files, and how to handle module dependencies. By understanding the nuances of ES6 modules and TypeScript, developers gain a powerful tool for building modular and maintainable codebases. Through hands-on examples and best practices, readers will learn how to leverage ES6 modules effectively to streamline development workflows and enhance code maintainability.
Leveraging Module Resolution Strategies
Module resolution is the process by which TypeScript determines the location of modules in a project, allowing developers to reference modules using relative or absolute paths. In this chapter, we explore the various module resolution strategies supported by TypeScript, covering their syntax, usage, and common use cases. We discuss best practices for configuring module resolution in different project setups, including how to handle module aliases, how to resolve module conflicts, and how to manage third-party dependencies. By understanding the nuances of module resolution strategies, developers gain a powerful tool for building modular and maintainable codebases. Through practical examples and best practices, readers will learn how to leverage module resolution strategies effectively to streamline development workflows and enhance code maintainability.
Advanced Techniques for Module Loading
Module loading is a critical aspect of modern web development, especially in large-scale applications with complex dependency graphs. In this chapter, we explore advanced techniques for module loading in TypeScript, covering dynamic imports, code splitting, lazy loading, and module bundling. We discuss best practices for implementing dynamic imports and code splitting, including how to optimize module loading performance and how to handle module dependencies. By understanding the nuances of module loading techniques, developers gain a powerful tool for building modular and maintainable codebases. Through hands-on examples and best practices, readers will learn how to leverage advanced techniques for module loading effectively to streamline development workflows and enhance code maintainability.
Dynamic Imports and Code Splitting
Dynamic imports allow developers to asynchronously load modules on-demand in TypeScript, improving application performance and reducing initial bundle size. In this chapter, we explore how dynamic imports can be used for code splitting, lazy loading, and implementing advanced loading strategies. We discuss best practices for implementing dynamic imports and code splitting, including how to optimize module loading performance and how to handle module dependencies. By understanding the nuances of dynamic imports and code splitting, developers gain a powerful tool for building modular and maintainable codebases. Through hands-on examples and best practices, readers will learn how to leverage dynamic imports and code splitting effectively to streamline development workflows and enhance code maintainability.
Integrating Third-Party Libraries with TypeScript
Third-party libraries play a crucial role in modern web development, providing pre-built solutions for common tasks and functionalities. In this chapter, we explore best practices for integrating third-party libraries with TypeScript, including how to install and configure type definitions, how to create custom type definitions, and how to handle type compatibility issues. We discuss how to integrate popular third-party libraries such as React, Angular, and Vue.js with TypeScript, covering common use cases and best practices. By understanding the nuances of integrating third-party libraries with TypeScript, developers gain a powerful tool for building modular and maintainable codebases. Through hands-on examples and best practices, readers will learn how to leverage third-party libraries effectively to streamline development workflows and enhance code maintainability.
Best Practices and Patterns for Advanced TypeScript Development
In this concluding chapter, we summarize the key concepts and techniques covered throughout the book and discuss best practices and patterns for advanced TypeScript development. We explore common design patterns and architectural principles for building modular and maintainable codebases, covering topics such as code organization, error handling, performance optimization, and code quality. By understanding the nuances of advanced TypeScript development practices and patterns, developers gain a deeper understanding of how to build robust and scalable software architectures. Through hands-on examples and best practices, readers will learn how to apply advanced TypeScript development practices and patterns effectively to streamline development workflows and enhance code maintainability.